So you’ve heard of Javascript. You’ve probably heard that Javascript is in-demand, or that it’s one of the top 5 skills you should learn to get hired as a web developer, or that it’s one of the most popular programming languages in use today. So you’re probably asking yourself the following questions: “What is Javascript used for exactly? And…should I learn Javascript?”
Maybe you’re an experienced programmer looking to grow your repertoire with a new skill, or maybe you’re a complete beginner hoping to pick up your first language. Either way, there’s no doubt that Javascript is a valuable tool, and that learning how to use it will make you more hirable.
What is Javascript Used For?
Javascript is a lightweight, high-level scripting language that is primarily known for making websites dynamic. Put simply, this means that
- Javascript does not use up a lot of memory or CPU and is easy for humans to understand
- Javascript can run on many different types of machines
- Javascript does things like allow you to click a button on a website
But front end web programming is just one application of Javascript. Nowadays, JS is also used to program native mobile apps, and on the backend as a server-side language. This is partly why it has become so popular, and what makes it a very valuable skill to learn.
Front End Web Development
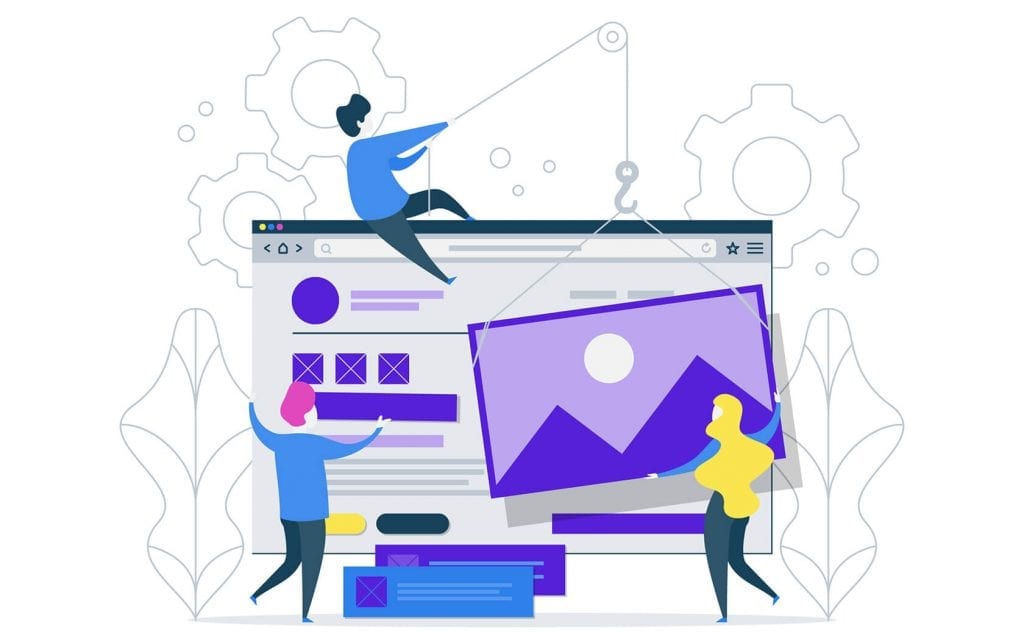
The front end is what you see when you visit a website in your browser. It’s the words you read, the images you view, the buttons you click, and the forms you fill in. Most of the time, it is made up of 3 basic components: HTML, CSS and Javascript.
HTML is the bones of a webpage. It tells the page what to show and where to put it. Want to render an image? Use an HTML <img> tag. Want a paragraph of text under that image? Use an HTML <p> tag.
<img
src="https://www.boredpanda.com/cute-kittens/?media_id=535581"
class="kitten"
/>
<p> Look at this cute picture of an adorable kitten. Awwww! </p>
CSS is the skin of the page. It tells the page how the HTML elements should look. Maybe you want your image to be 800px wide and 600px high – you will use CSS for that.
.kitten {
width: 800px;
height: 600px;
}
Javascript is the brains of the page. It tells the page how to react to user interaction. Do you want the image to expand when someone hovers their mouse over it? Javascript will help you do that.
const image = document.getElementByClassName('kitten');
const expandImage = () => {
image.style.width = 1200;
image.style.height = 1000;
}
image.onMouseOver(expandImage());
Front end Javascript also handles animation, form validation and fetching data from the server. When you visit a web page, a piece of JS code asks a server to send the information that you should see.
For example: say you log in to your favorite photography website, where you follow a few different photographers. Javascript sends your login information to a server, and the server sends back information about what images you should see in your feed. Javascript organizes this information and puts it into the HTML <img> tags.
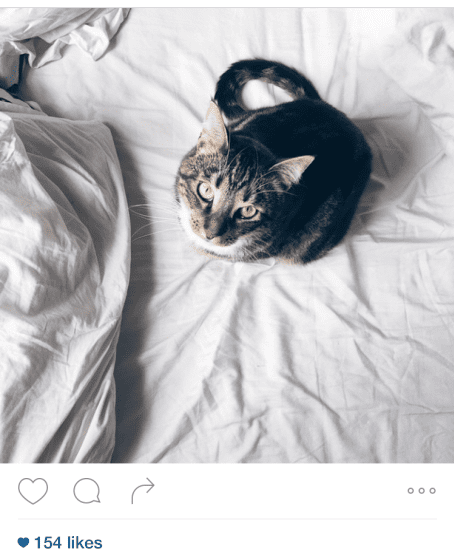
Now let’s say you “like” a picture. Javascript not only listens for when you click the “like” button, but it also sends all the information about the image you liked back to the server and updates the style of the button so you can see that it has been clicked.
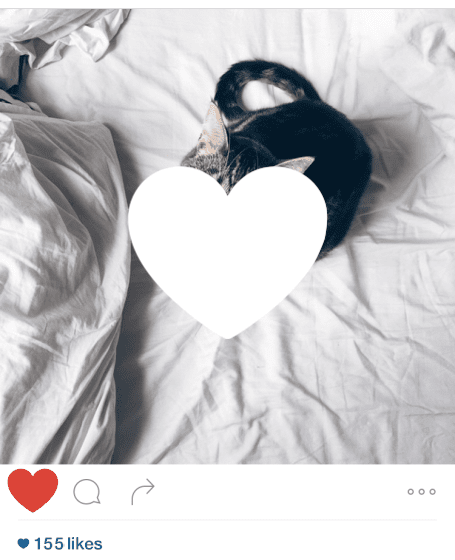
Neat! But that’s not all…
Native Mobile App Development
It’s not 1995 anymore, so unless you’ve been living under a rock, you’re probably viewing your “favorite photography website” as an app on your phone.
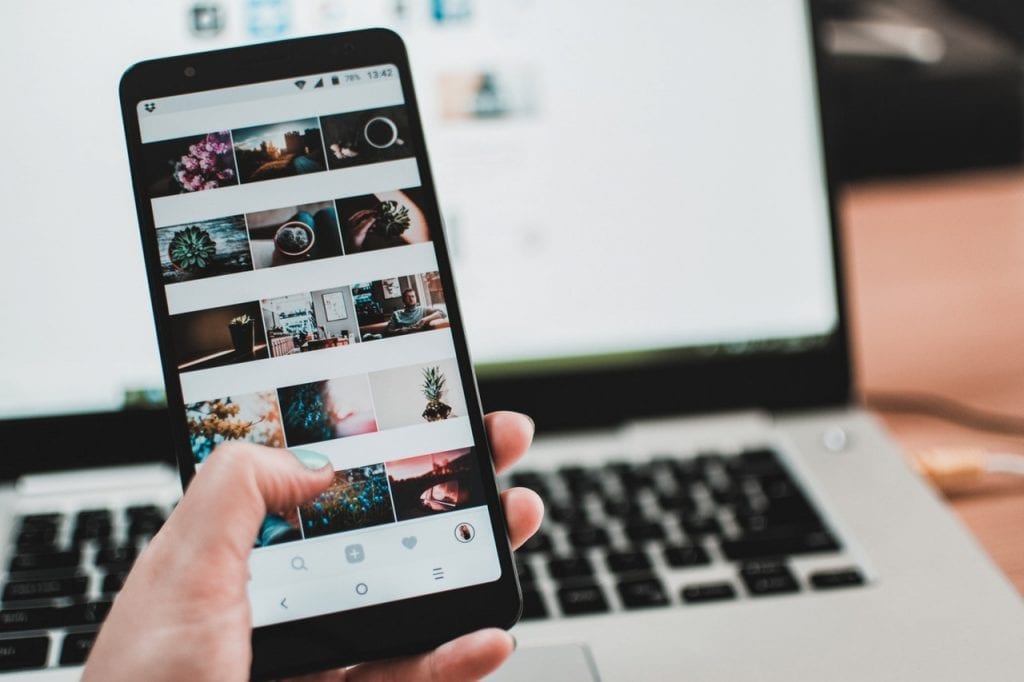
Mobile app development is different from web app development. Web apps and websites are basically small programs that run inside the browser. Mobile apps are programs that run on a phone’s operating system. It’s like the difference between installing Photoshop on your computer vs using an online photo editor.
This means that the languages you use to write those programs might have to be different. The operating system on an iPhone is not the same as the OS on an Android phone, and so the app you wrote for iPhone might not run properly on Android. You might even have to write a whole separate version of your app to work on Android.
Using Javascript libraries like React Native, Cordova and Nativescript, however, you can write code in Javascript that compiles to native mobile code. This means that the JS library will translate your JS code into the correct low-level language for the device it is running on. So you can write just one version of your app that will work everywhere: in the browser, on an iPhone, on Android, Pixel, etc. There are some downsides to writing apps this way, but you gain a lot in areas like the speed of development and flexibility.
Back End App Development
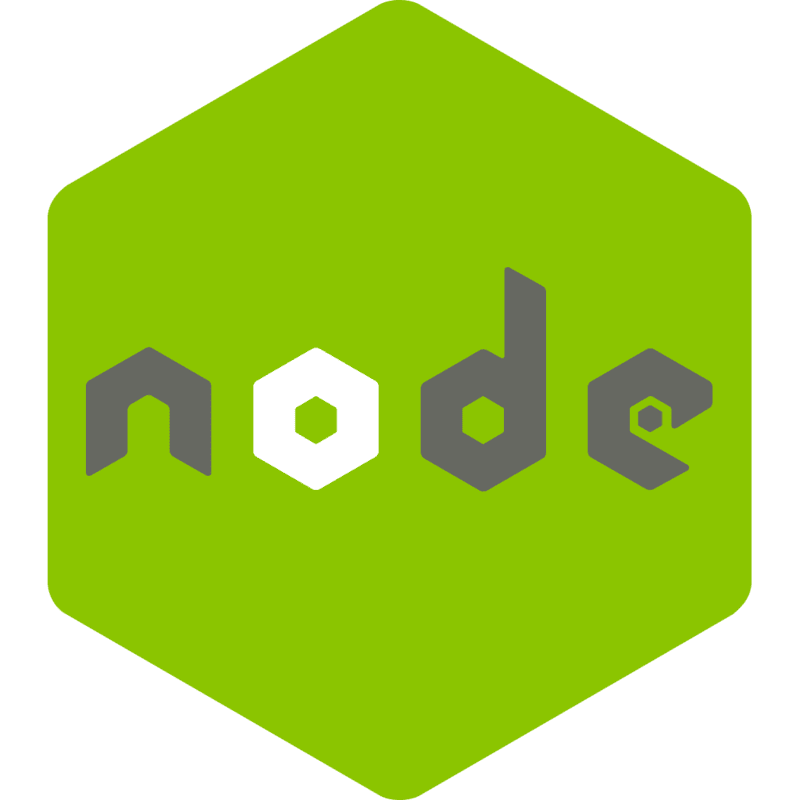
NodeJS is a Javascript runtime environment.
Cool, you’re thinking. WTF does that mean?
A runtime environment is just code that runs other code. Your web browser is a runtime environment: it is a piece of software that runs the code that displays websites and web apps. NodeJS is kind of the same thing, but it runs on servers. It uses something called the V8 Javascript runtime engine to translate Javascript code into faster, low-level machine code and runs it directly on that machine. The V8 engine is written in C++, and it also allows you to write C++ code that can hook directly into your Javascript.
The cool thing about NodeJS is that it’s perfect for programming web servers because it is non-blocking. That means that Node can run many different requests from many different users at once, without having to wait for some requests to finish before starting new ones.
Should I Learn Javascript?
“Okay,” you’re saying, “I get it! Javascript is useful. But should I learn it?”
That, grasshopper, is a question only you can answer. No one can tell you what to learn (unless you are in elementary school, in which case…this article is maybe above your paygrade.) But I can provide some examples of people who would definitely benefit from learning Javascript.
You Want to Become a Web Developer
This article should make it pretty clear that Javascript is all over the web. Even if you want to become a Ruby on Rails developer, there’s a very good chance that you are still going to need to use Javascript for some things.
You Want to Learn Your First Programming Language
Because Javascript is a high-level language, it is relatively easy to understand compared to low-level languages. You don’t need to worry about things like memory allocation, garbage collection, typing, threading and lots of other complicated stuff. Also, because it is so popular, there are tons and tons and tons of resources out there to help answer your questions. This means Javascript is an awesome language to start with if you want to get your feet wet in the coding pool.
You’re a Programmer Looking to Add Another Tool to Your Belt
Look man, whether you love it or hate it, Javascript is here to stay. If you already program in another language (or several), picking it up shouldn’t be too hard, and will make you more versatile, hirable and future-proof. Even if you don’t end up using it in your day-to-day life, knowing how to converse effectively with people who do use it will make debugging faster, and communication easier.
Have you tried to learn Javascript? What are your thoughts on JS? Are you a newbie coder, a career-changer, or a seasoned pro? Let us know in the comments below, and don’t forget to check out some of our other articles on JS frameworks like best ReactJS courses, AngularJS courses and TypeScript courses.